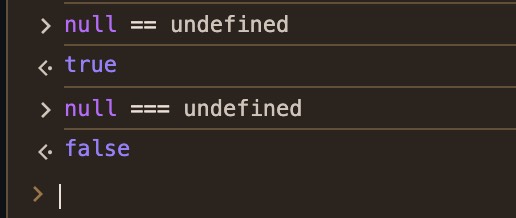
Abstract:
This article discusses the differences between two distinct values in JavaScript, null
and undefined
. These values often confuse beginners as both represent the absence of a value. This article aims to explain the fundamental concepts, key differences, and appropriate use cases for each. Furthermore, it explores their behavior with comparison operators, data types, and provides relevant examples to aid understanding.
Introduction:
JavaScript is a highly flexible programming language but can be perplexing for newcomers, especially when dealing with null
and undefined
. While both signify the absence of a value, they have different meanings and use cases. A clear understanding of these differences is essential to write efficient code and avoid hard-to-detect bugs.
Differences Between null
and undefined
:
-
Definition:
undefined
: A default value assigned by JavaScript to variables that are declared but not initialized or to non-existent object properties.null
: An explicit value used to indicate that a variable has been intentionally set to have no value or to be empty.
-
Data Type:
undefined
: Its type isundefined
.null
: Its type isobject
, a legacy bug in JavaScript.
-
Usage:
undefined
: Commonly used by JavaScript for uninitialized variables or missing object properties.null
: Typically used to explicitly clear a variable or to indicate an empty or non-existent object.
-
Comparison:
undefined == null
: Evaluates totrue
as both are treated as "empty values."undefined === null
: Evaluates tofalse
because they have different data types.
Use Case Examples:
-
Uninitialized Variable:
let x; console.log(x); // Output: undefined
-
Assigning
null
to a Variable:let y = null; console.log(y); // Output: null
-
Comparison:
console.log(undefined == null); // Output: true console.log(undefined === null); // Output: false
-
Non-Existent Object Property:
const obj = {}; console.log(obj.property); // Output: undefined
Conclusion:
Although null
and undefined
both signify the absence of a value in JavaScript, they serve different purposes and should be used in different contexts. Understanding these distinctions is crucial for writing more robust JavaScript code and avoiding logical errors, especially when performing comparisons or type checks.